Create a Fibonacci Series in Python using for Loop, Recursion & Dynamic Method
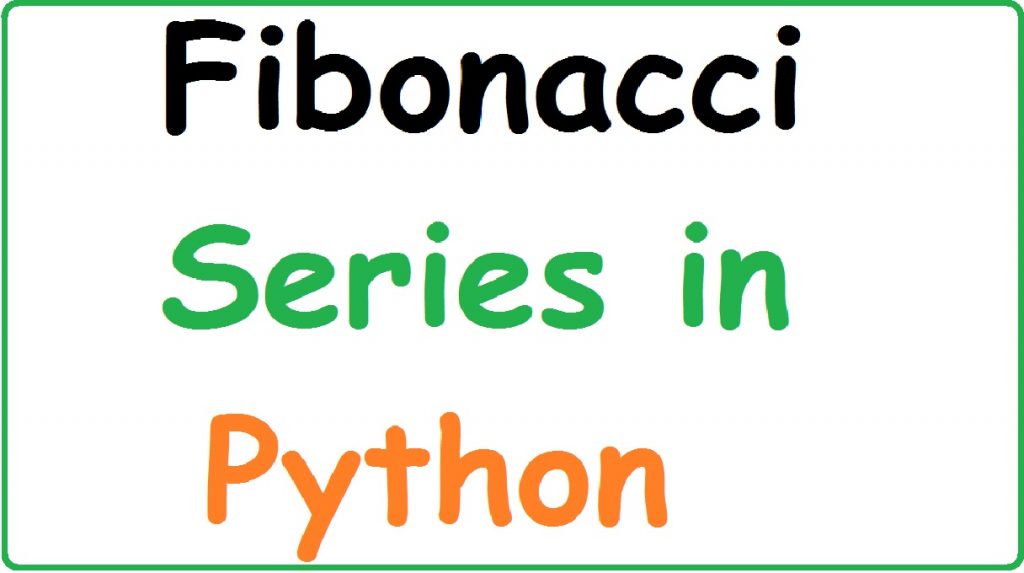
Python Program for Fibonacci Series using for Loop, Sequences, Numbers 2020
Fibonacci Series:
Fibonacci is a series of numbers developed by the Italian mathematician. It is a sequence of the numbers from 0 and 1 and followed by the other numbers.
The other numbers are obtained by adding the preceding numbers. In this topic, we will show you how to write a Fibonacci series in Python with a simple program.
Working Principle of Fibonacci Series in Python
The Fibonacci series is a sequence of numbers that starts from 0, 1 and followed by the other numbers. The computer only reads only binary numbers i.e. 0 and 1 so you get the other numbers by adding the preceding numbers. The equation is an example of the working principle of the Fibonacci series.
If we want 0 and 1 so 0+1=1
Example of Fibonacci series: 0,0,1,1,2,3,……
Program of Fibonacci series in Python:
The number 3 output get by adding the two numbers preceding i.e. 1+2=3, 2 get by adding 1+1=2.
So the loop continues until you add the stop code in the program.
And if we take the number as function then the code is as follows:
Fn=Fn-1+Fn+1 and the seed values is given by the F1=1 and F0=0
Fibonacci series program in python using for loop
We are creating a Fibonacci series loop using Python code editor. Python has specialized loops that execute the code multiple times.
# Enter number of terms needed #0,1,1,2,3,5.... a=int(input("Enter the terms")) f=0 #first element of series s=1 #second element of series if a<=0: print("The requested series is",f) else: print(f,s,end=" ") for x in range(2,a): next=f+s print(next,end=" ") f=s s=next
Program of Fibonacci Series Using Functions (Recursion Method)
Fibonacci series in python using functions (Recursion Method)
In Recursion method we are discussed that we use the term ‘n’ to obtain the output. This function is known as Recursive Function.
The program as follows:
# Function for nth Fibonacci number def windowstrends(num): if num<0: print("Incorrect input") # First Fibonacci number is 0 elif num==0: return 0 # Second Fibonacci number is 1 elif num==1: return 1 else: return windowstrends(num-1)+windowstrends(num-2) print(windowstrends(9))
Program of Fibonacci Series Using Dynamic Method:
Dynamic Method is also used to create a Fibonacci series loop. In this program, we take the numbers 0 and 1. The program as follows:
# Fibonacci Series using Dynamic Programming def windowstrends(n): # The starting two fibonacci nubers are 0 and 1 Fibseries = [0, 1] while len(Fibseries) < n + 1: Fibseries.append(0) if n <= 1: return n else: if Fibseries[n - 1] == 0: Fibseries[n - 1] = windowstrends(n - 1) if Fibseries[n - 2] == 0: Fibseries[n - 2] = windowstrends(n - 2) Fibseries[n] = Fibseries[n - 2] + Fibseries[n - 1] return Fibseries[n] print(windowstrends(9))